Dynamic Programming: Solving the Staircase Problem
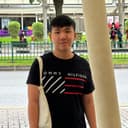
Introduction
In this blog post, we'll explore the dynamic programming approach to solving the classic "staircase problem" using Python. The staircase problem involves finding the number of distinct ways to climb a staircase of n
steps, with the possibility of taking 1 or 2 steps at a time.
Problem Statement
Given a staircase with n
steps, find the number of distinct ways to climb it, where at each step you can either take 1 or 2 steps.
Overlapping Subproblems
- Suppose we want to find the number of distinct ways to climb a staircase of n steps.
- If we consider the last step taken, it can either be a single step from n-1 or a double step from n-2.
- Therefore, the number of ways to climb n steps is the sum of the number of ways to climb n-1 steps and n-2 steps.
- This property allows us to break down the problem into smaller subproblems (climbing n-1 and n-2 steps) and combine their solutions to find the solution to the original problem (climbing n steps).
Optimal Substructure
- Suppose, for the sake of contradiction, that is not an optimal solution to .
- Let be a solution to that is not optimal, meaning there exists another solution that is better than .
- Consider a staircase with n steps. By assumption, is not an optimal solution, so there exists another solution that is better than .
- Analyzing the last step of . There are two cases:
- Case 1: The last step of is a single step. In this case, we can remove this step to get a solution for . Call this solution .
- Case 2: The last step of is a double step. In this case, we can remove these two steps to get a solution for . Call this solution .
- By the induction hypothesis, and are optimal solutions for and , respectively.
- Now, we can construct a new solution for by combining the optimal solutions for and :
- By construction, is at least as good as , since we've combined optimal solutions for the subproblems. This contradicts our assumption that is better than .
- Therefore, our assumption that is not an optimal solution to must be false, and we conclude that T(n) has an optimal substructure.
Recurrence Formula
The recurrence formula for the staircase problem is given by:
Dynamic Programming Approach
Dynamic programming is a technique used to solve problems by breaking them down into simpler subproblems and solving each subproblem only once. We'll use dynamic programming to efficiently solve the staircase problem by building up solutions to smaller subproblems.
Let's define a function count_ways(n)
that takes the number of steps n
as input and returns the number of distinct ways to climb the staircase.
We use an array of size to store the number of ways required for each step.
def count_ways(n):
# Base cases
if n == 0:
return 1
elif n < 0:
return 0
# Initialize an array to store solutions to subproblems
dp = [0] * (n + 1)
# Base cases
dp[0] = 1
dp[1] = 1
# Build up solutions to larger subproblems
for i in range(2, n + 1):
dp[i] = dp[i - 1] + dp[i - 2]
# Return the solution to the original problem
return dp[n]
# Example usage
n = 5
print(f"Number of distinct ways to climb {n} steps: {count_ways(n)}")